
Let's begin by creating a simple constructor function with a head pointion to null. To implement this data structure we'll use prototypal inheritance using JavaScript. We can use the pointer to traverse the elements in the node. The first node is known as the head node. Linked ListĪ linked list is a linear collection of data elements, called nodes, each pointing to the next node. However, if you don't care about immutability or persistence, there is very little downside to including a tail pointer.

Tail pointers break both of those properties.

Also, the last node in the linked list can be identified. In fact, in functional programming languages, this usage is ubiquitous. You have to start somewhere, so we give the address of the first node a special name called HEAD. I encourage you before you begin to try to implement these data structures by yourself first. Linked lists are very commonly persistent and immutable. A class Stack is created which contains some of the Stack methods such as push(), top(), pop() etc.
LINKED LIST STACK HEAD HOW TO
In this blog post we'll discuss how to implement a stack, queue and linked list using JavaScript. The top element of the stack is: 7 The stack element that is popped is: 7 The stack element that is popped is: 9 The top element of the stack is: 3. For the sake of this blog article we'll just discuss linked lists, queues and stacks using JavaScript This data structure makes it possible to implement a stack as a singly linked list and as a pointer to the top element. Note: Head is not a separate node but it is a reference to the first node. Some other common data structures include trees, tries & graphs, heaps, array lists and hash tables. Last link carries a link to null to mark the end of the list. Depending on the implementation a stack may, or may not, keep track of the previous value. The linked list can be written as a circularly linked list, double linked list or singly linked list.
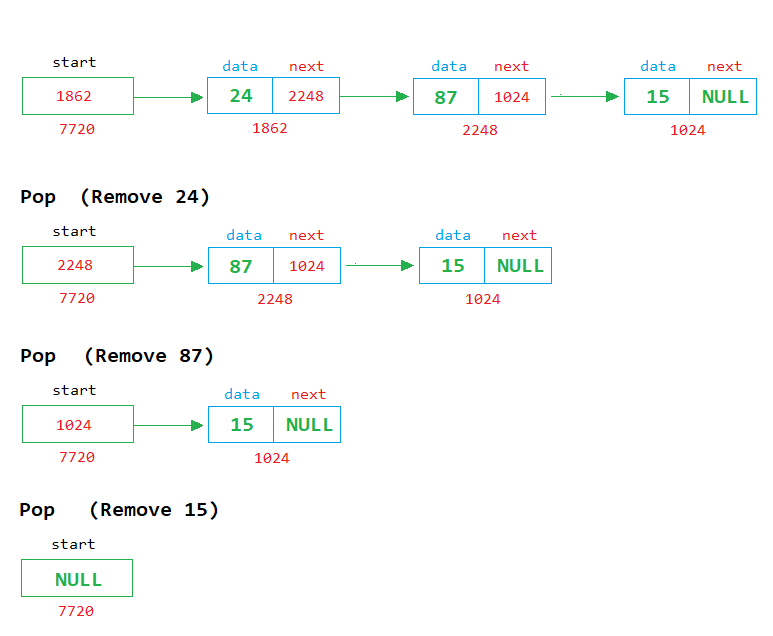
On the other hand a stack and queue keeps track of the top value, size and previous value in most cases.Įach implementation of these data structures may differ slightly. In the linked list one pointer is used to keep track of the head and another keeps track of the next value. All three hold a collection of elements and can be traversed. Insertion of Values in Linked List head fi, in which case the node we are adding is now both the head and tail of the list or we simply need to append our. The linked list, stack and Queue are some of the most common data structures in computer science. How To Create A Linked List, Stack and Queue in JavaScript from tabulate import tabulate class Node: def init(self, value): self.data value self.next None Next Node's address self.prev None Previous Node's address class DoublyLinkedList: def init(self): self.head None Empty linked list self.tail None Last node in the list self.n 0 Number of nodes def len(self): return.
